The lzRead Deep Dive
Overview
By default, smart contracts lack access to the state of other blockchains and have only limited access to the historical state of their own chain. Ideally, smart contracts on every blockchain could easily access all data; whether that is cross-chain state, historical state, and Web 2.0 data sources (e.g., REST and GraphQL APIs), all through one interface. Additionally, the interface should be secure, where queries are carried out through an immutable, censorship-resistant protocol with application-owned security configurations. This architecture would provide smart contracts with a holistic view of global data with bare minimum onchain trust assumptions and maximum offchain security sovereignty.
LayerZero’s solution for global data visibility includes two key contributions:
- Blockchain Query Language (BQL): a framework that defines a declarative method for smart contracts to query both on-chain and off-chain data, current and historical, without being tied to any specific protocol implementation.
- lzRead: the LayerZero-specific implementation that operationalizes BQL. Leveraging the LayerZero protocol alongside a new Message Library, lzRead is a smart contract querying interface that forwards encoded queries to decentralized verifier networks (DVNs), enabling data retrieval from both on-chain and off-chain sources.
While BQL provides the broad conceptual approach for querying data, lzRead offers a flexible, cost-efficient, and low-latency solution that can adapt to evolving encoding methodologies without necessitating a complete replacement of its underlying components.
This report provides a comprehensive overview of lzRead, covering three key sections: background, BQL, and lzRead. By the end, you'll understand what BQL is, why lzRead is an essential developer tool in the fragmented blockchain landscape, and how it works together to enable secure, low-latency, and cost-efficient querying.
Background
Push messaging is where a smart contract on one chain writes (i.e. updates the state) to a smart contract on a different chain. While this works for many use-cases like token bridging and cross-chain smart contract calls, it is an inefficient method for a smart contract to query data from another chain – a method often referred to as pull messaging.
Querying data by using push-messaging requires multi-step messages, which increases the total latency and gas costs beyond what is necessary. For example, a smart contract on Base retrieving the previous owner of a Non-Fungible Token (NFT) on Ethereum would require one message sent from Base to Ethereum to retrieve the data, another push message from Ethereum back to the smart contract on Base. This requires four total gas payments (paid twice) with latency across two pathways. Considering the historically high gas costs and finality requirements of Ethereum, it renders this method practically infeasible.
In a perfect world, the example above should be possible with bare minimum onchain gas and latency requirements; a total of one gas payment on the source chain for initiating the query and speed constrained only to how fast the offchain verifier can fetch the requested data. Furthermore, the ideal solution should be optimal, not only from a cost and speed point of view, but also in the type of data that can be sourced. A smart contract should be able to query data across all spatial and temporal dimensions (space and time) – onchain and offchain, current and historical. This is only possible via pull-messaging pattern without multi-step messages that touch many chains. What this enables is, for instance, a DEX on Ink would be able to query and aggregate onchain Uniswap v3 ETH/USDC pool data from Ethereum, Binance Smart Chain, and Optimism ("data chains") without ever submitting a transaction on those data chains.
Existing solutions to cross-chain querying via pull messaging pattern, including those in research and development, are constrained by the lack of a complete cross-chain mesh.
- For example, the solutions in the Ethereum community for cross-L2 state reading necessitates all the L2’s to access either the recent L1 state root, or the entire L1 state. This incurs problems, however. For example, the RemoteStaticCall solution requires infrastructure modifications, introduces latency, and relies on secure verification, while L1SLOAD depends on L1 node access, incurs high gas costs, and adds RPC latency. Both solutions, though functional, highlight the need for a more seamless and standardized cross-chain query mechanism.
- Oracles, whether push or pull, enable smart contracts to retrieve data, but they are often not permissionless solutions and are limited to the chains they support, and the data the providers source. Furthermore, they offer no guarantees of data integrity, relying on a "trust, don’t verify" model. As a result, smart contract developers lack a permissionless method, with support on all chains, to pull arbitrary on-chain or off-chain data from any source.
These problems indicate the need for a more optimal solution, one that includes a framework for defining onchain queries (BQL), and a protocol to execute said queries (lzRead) across the entire blockchain ecosystem.
Blockchain Query Language (BQL)
The Problem
Smart contracts lack a universal, standardized way to perform arbitrary data queries, especially for data outside their own blockchain. As discussed above, any mechanism for retrieving data beyond the contract’s local state tends to be limited either spatially (confined to a specific blockchain or data source) or temporally (confined to recent history). On an EVM-based blockchain (like Ethereum), a smart contract can expose read-only functions (view/pure in Solidity) to return on-chain data from its own state or other contracts’ current state. However, contracts cannot directly fetch off-chain or cross-chain information without some other infrastructure. Even on their own chain, they have very limited historical awareness – for instance, Ethereum contracts can only access the last 256 block hashes natively, and no built-in ability to query older block data exists.
These challenges grow even more complex across different execution environments. Each VM has its own constraints: an Ethereum (EVM) contract and a Solana program (SVM) handle data access differently (global vs. account-based addressing, synchronous calls vs. predefined accounts), but neither can escape the fundamental limitations on data querying without help from off-chain systems. In short, today’s smart contracts are inherently limited in where and when they can fetch data.
The Solution: BQL
The Blockchain Query Language (BQL) provides a flexible framework for structuring queries across diverse blockchain execution environments. Specifically, it enables smart contracts to query data using a structured set of parameters, ensuring consistency despite differences in execution environments, consensus finality, and timestamp precision. BQL supports various retrieval methodologies, including direct block number queries, timestamp-based selection, and processing (compute) functions like MapReduce to enhance its utility.
BQL is universal, identical across all programmable blockchains regardless of their architectural differences (e.g., EVM vs. altVMs), and comprehensive, as it supports queries spanning all data sources (spatial) and time periods (temporal). Queries, structured according to BQL semantics, that target blockchain data has a range of possibilities:
- Real-Time: Fetch current data at the latest block (e.g., the most recent ETH/USDC price from a Uniswap v3 pool).
- Historical: Retrieve past data from any recorded history (e.g., the price of ETH/USDC 100 blocks ago).
- Point-in-Time: Capture specific data snapshots at a given block (e.g., the total supply of USDC on Ethereum at block X).
- Range-Based: Aggregate data over a block interval (e.g., calculate a TWAP over 100 blocks).
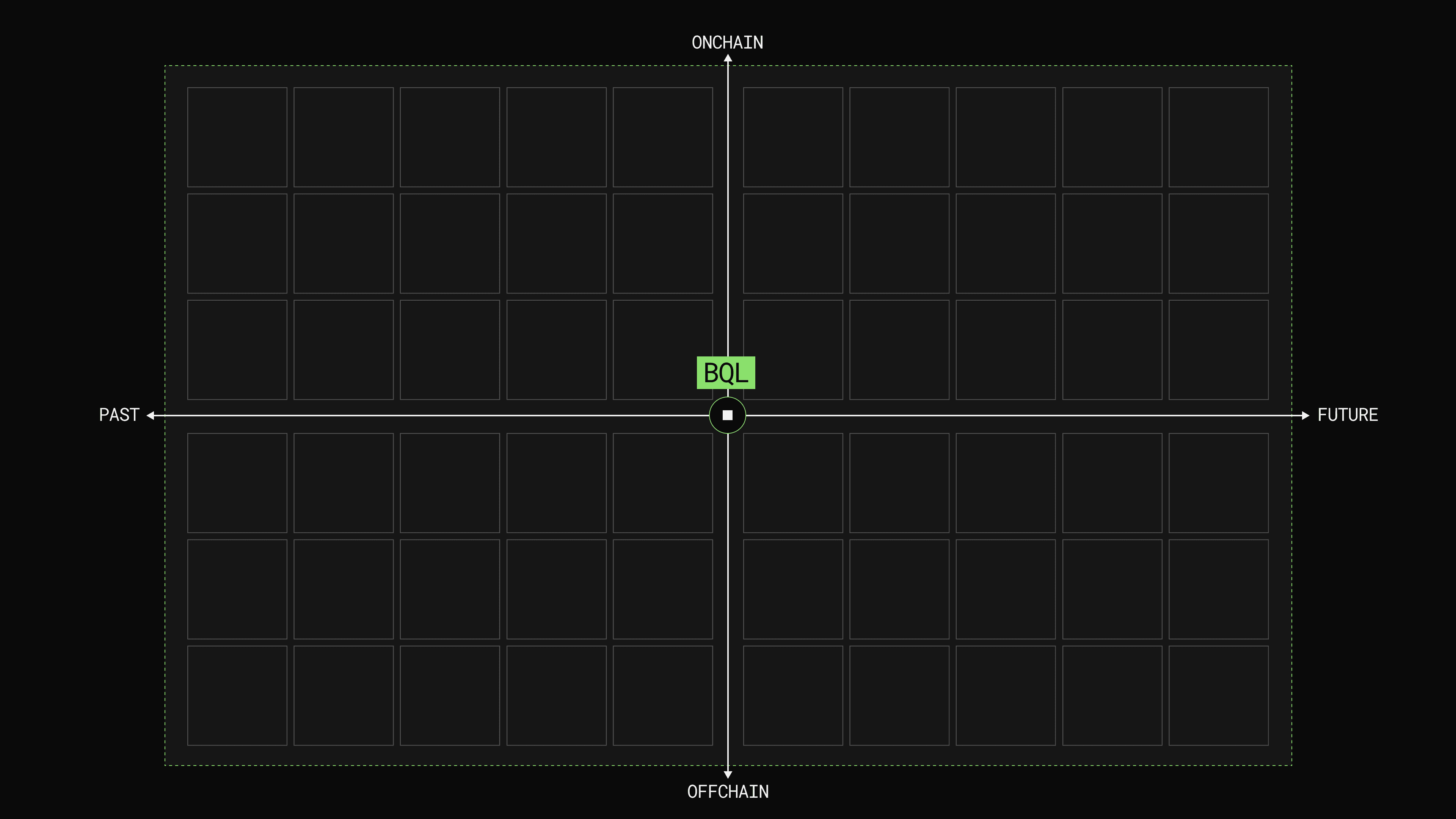
Design
Each BQL query has three components: Read, Compute, and Version. Query and Compute have multiple parameters.
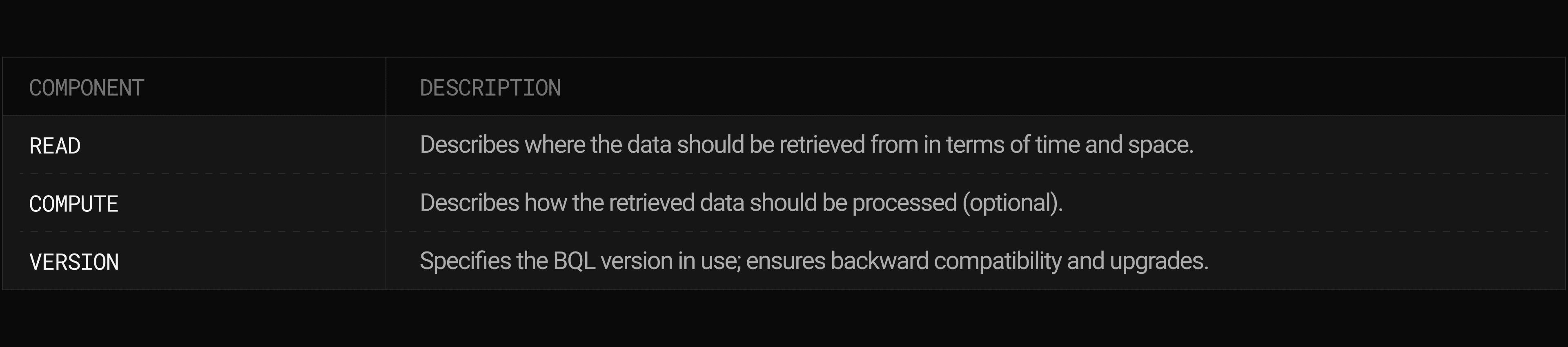
Table 1: Each BQL query has three parts – Read, Compute (optional), and Version.
The Read component includes several parameters that are important to discuss in detail beyond what is outlined in Table 2. The targetEID specifies the data source, where each chain is giving an ID, but an ID can also include external web2.0 sources. If the smart contract chooses to specify the blockNum then the query points to the designated block number in time. If the blockTimestamp is chosen, a convention must be applied to resolve the situation where the timestamp does not align perfectly with a target blockchain’s block production timing. This can be due to the differences between blockchains block times, consensus mechanism delays, finality requirements, or lack of millisecond precision. The preferred resolution convention is to apply the greater than or equal to property, which guarantees that the data retrieved is at least as recent as the specified timestamp. If the convention allows a block to be selected slightly before the target time, there is a risk of retrieving outdated or non-final data, which would not accurately reflect the state intended by the timestamp.
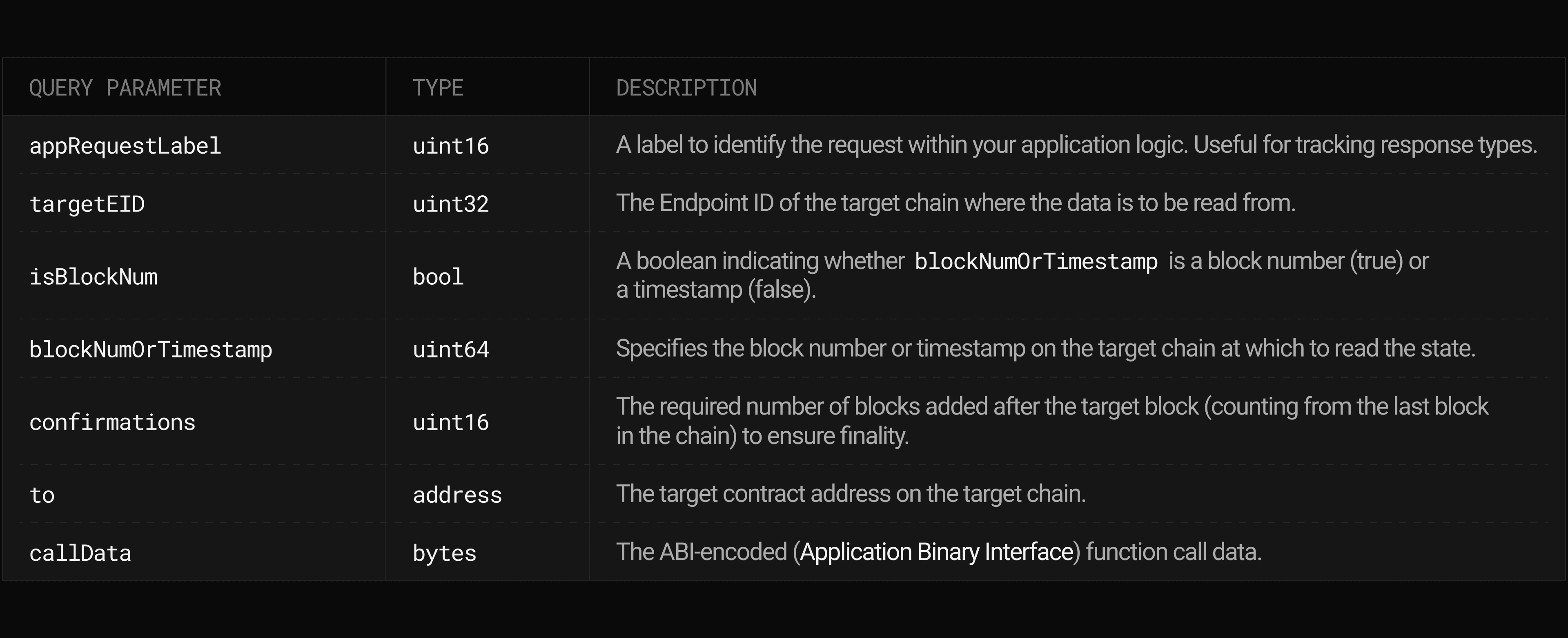
Table 2: The Query component describes where data should be fetched from. It has seven parameters that specify the endpoint, the function call payload and the location on the target chain.
The Compute component specifies the processing methods applied to retrieved data. BQL supports the familiar MapReduce abstraction with two operations, Map and Reduce, which can be applied individually or in combination.
- Map: parameter that organizes data from various sources into key-value pairs. For example, Map could retrieve the ETH/USDC price from Uniswap v3 on Ethereum and the ZRO/USDC price from Uniswap v3 on Arbitrum, returning each in a single response structured like {"ETH": "USDC_price"} and {"ZRO": "USDC_price"}.
- Reduce: parameter that aggregates and summarizes these pairs based on their keys to produce a final result. In comparison to the Map output, the Reduce output would look like "prices": {"ETH": "USDC_price", "ZRO": "USDC_price"}. Accordingly, this function can be especially useful for applications that require summarized datasets, such as calculating averages, sums, or other metrics before returning the final processed data to the source chain.
Furthermore, BQL provides four distinct settings within the Compute function to address different processing needs:
- Map Only: Applies transformations to each query result individually, ensuring consistency across datasets.
- Reduce Only: Aggregates responses to produce a consolidated output, suitable for summary results.
- MapReduce: Combines mapping and reducing processes, allowing for more complex data processing tasks.
- None: Retrieves raw data directly, executing queries without additional computation.
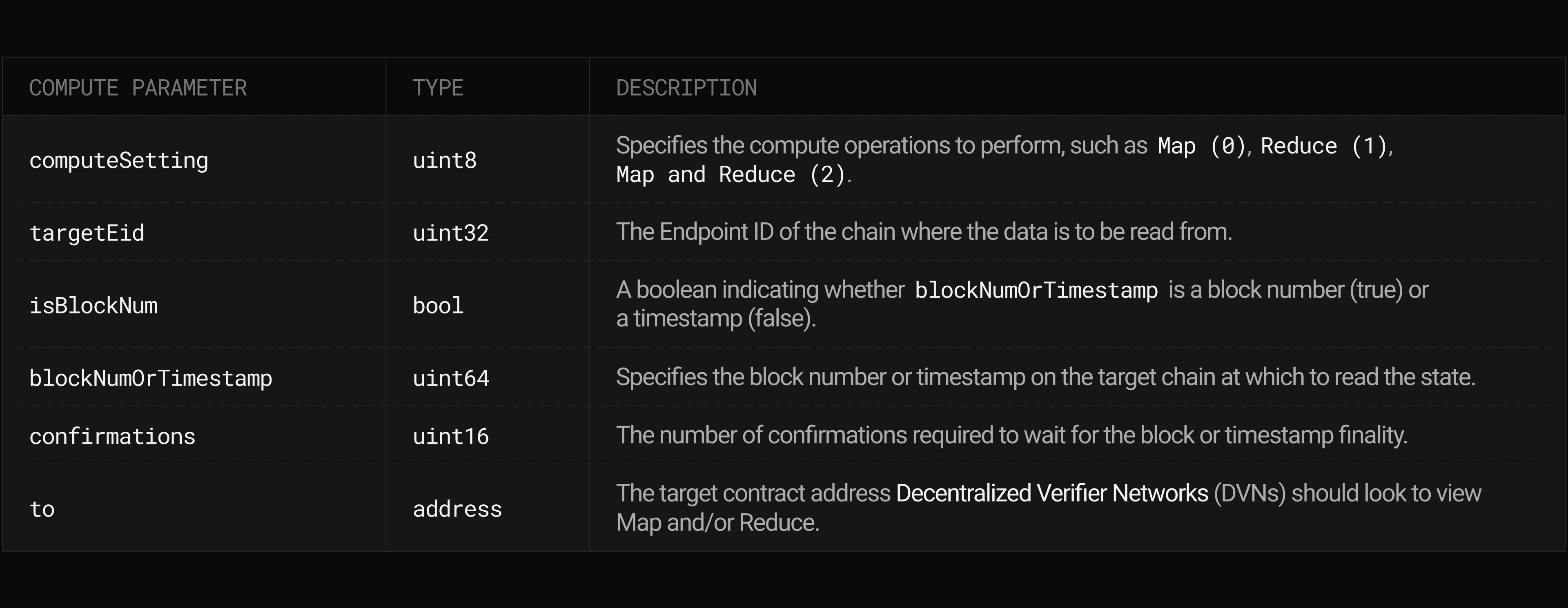
Table 3: The Compute component allows applications to specify MapReduce transformations on the retrieved data. It is optional and can be bypassed by setting computeSetting to none.
Requirements for BQL implementations
BQL can be implemented as an extension to a blockchain virtual machine (VM) with minimal overhead. To support BQL, a blockchain VM simply needs to be able to encode bytes and emit those bytes in an event, which the vast majority of blockchains support. Additionally, a verifier, or set of them, are needed to pick up encoded requests, fetch the corresponding data, compute it (optional), and return it back to the requesting application – more on this in the lzRead section. Overall, BQL's minimal implementation requirements is a key reason why it can universally support any blockchain.
lzRead
Overview
lzRead is the execution engine for BQL queries. It applies BQL’s properties in being universal and comprehensive in an onchain implementation that can be used by smart contracts, while leveraging LayerZero v2 protocol infrastructure, to query any data from any chain over any period of time. lzRead is built as part of the LayerZero v2 protocol, as such, it does not introduce any new trust assumptions outside of an immutable Read Message Library. To simplify the primitive’s structure we divide it into three layers: semantics, protocol, and processing. The components within each layer play a role in ensuring application’s query requests and responses are carried out securely, quickly, and cheaply.
Design Goals
Fast
The lzRead request-response pattern (AA message pattern) eliminates unnecessary roundtrips, making execution more efficient by avoiding multi-step exchanges like those in the ABA pattern. It reduces request-response latency to just the finality time of the source chain, along with one-way verification and execution. This setup means that as single-slot finality becomes standard, and DVN infrastructure advances, requests could eventually be fulfilled in the block immediately following the request.
ABA Message Pattern Latency = source chain finality (A) + verification (AB) + execution (AB) + source chain finality (B) + verification (BA) + execution (BA)
This simplifies to waiting for finality on two chains, followed by verification and execution in two separate pathways. On the other hand, lzRead’s latency is the following:
AA Message Pattern Latency = source chain finality (A) + verification + execution
Cheap
With the AA message pattern, gas fees are incurred only on the source chain for both the request and the response. Since there is no message roundtrip as in the ABA pattern, costs are significantly lower. Additionally, verifiable entities (DVNs) trusted by the application securely handle data retrieval and aggregation, reducing on-chain computation expenses while maintaining data integrity.
The costs for the ABA and AA (lzRead) message patterns are simplified in the following expressions:
ABA Message Pattern Cost = source chain gas cost (A) + source chain gas cost (B)
AA Message Pattern Cost = source chain gas cost (A)
Secure
lzRead was designed to minimize the amount of trust assumptions outside of the LayerZero protocol. As such, lzRead was built as part of the LayerZero protocol (messages still go through the Endpoint), with ReadLib as the only component. Also, due to the round trip (AA) message pattern, a mechanism needed to be put in place to ensure the integrity of smart contract queries. To prevent reorg inconsistencies, each cmdHash, emitted upon a request, is compared to the cmdHash included in the response. If they don’t match (e.g., because the response corresponds to a different chain state due to a reorg), the response is rejected. Designing lzRead in this way means there are very minimal additional trust assumptions placed on developers already using LayerZero.
Core Components
Overview
This section explains the core components of lzRead, structured across three distinct layers.
- Semantics Layer: defines the query logic and syntax used by developers through the BQL (section 2). It is modular from the lzRead protocol and extensible to support any blockchain with minimal requirements.
- Protocol layer: consists of the LayerZero v2 protocol with the addition of messaging channels and the Read Message Library (ReadLib). This layer ensures developers have flexible control over their queries, and that they are carried out in a secure manner.
- Processing layer: includes offchain infrastructure that is used for the LayerZero v2 protocol, with the difference in that the DVNs fetch data, and execute computations.
The modularity across these layers allows lzRead to inherit the universal semantics and comprehensive properties of BQL, while delivering a secure, fast, and cheap solution for querying data from any environment. In the following sections, we will break down the core components specific to lzRead and then walk through how a lzRead request and response works.
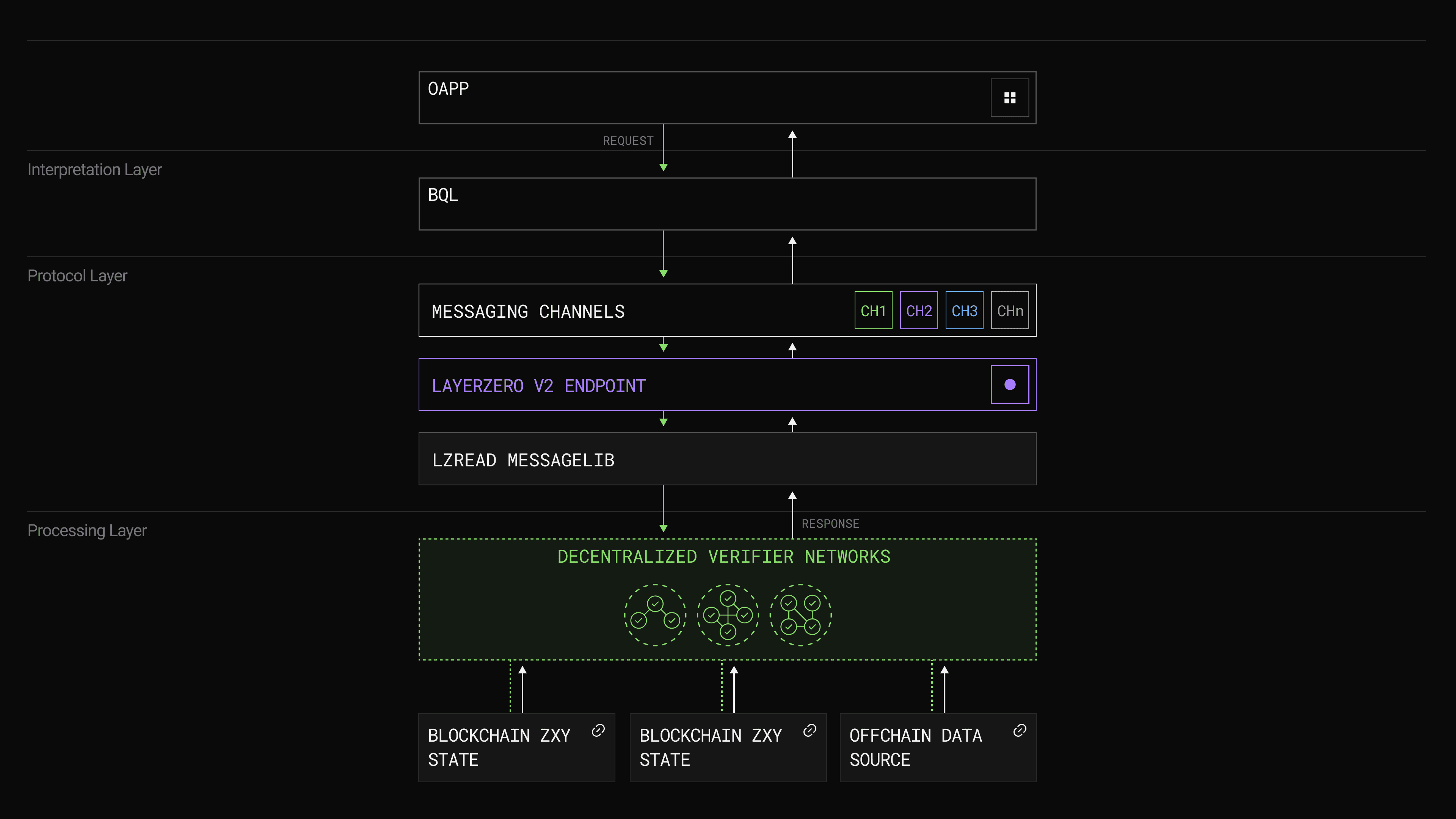
Semantics Layer
BQL: lzRead leverages BQL to enforce a defined query format while maintaining flexibility for future BQL versions. This ensures queries are structured, standardized, and securely executed within the protocol’s framework. BQL is implemented through the ReadCodec, enabling lzRead to access various types of on-chain data, including calldata, public state variables, and functions that do not modify blockchain state (i.e., view and pure functions).
Protocol Layer
The protocol layer itself can be split into three components:
1. Endpoint: The LayerZero endpoint is an immutable smart contract that provides a standardized interface for OApps to configure security settings and facilitate seamless message transmission. lzRead is built using the LayerZero endpoint, inheriting its security guarantees, immutability, and censorship resistance. This ensures that lzRead does not introduce any new trust assumptions beyond those already established by LayerZero.
2. Messaging Channels: Messaging channels provide a framework for applications to manage query types and configurations. Channels can be utilized by specifying a unique Channel ID, representing a logical pathway for a specific type of query. Developers use them for the following reasons:
- Different finality requirements (e.g., 1-block vs. 10-block confirmations).
- Different data sources or DVN sets (some DVNs might have specialized off-chain data coverage).
- Parallelizing requests to eliminate blockage (a high-latency historical query should not slow down a real-time price query).
lzRead supports millions of independent channels i.e., independent query configurations, allowing customization to meet developer’s specific requirements. They can be configured in many different ways, such as:
- Single channel, single source: Cost-efficient approach useful for simple queries. E.g., reading the holder of an NFT on a different blockchain.
- Single channel, multi-source: Aggregates data from multiple blockchains within a single channel. E.g., To fetch the price of a token across multiple blockchains.
- Multi-channel, single-source: Uses the same query, enabling redundancy for improved liveness and speed as potentially defective channels and slow DVNs chains do not bottleneck the process.
- Multi-channel, multi-source: Useful for applications that want the properties from (3) and the ability to perform diverse requests as outlined in the example from (1) and (2).
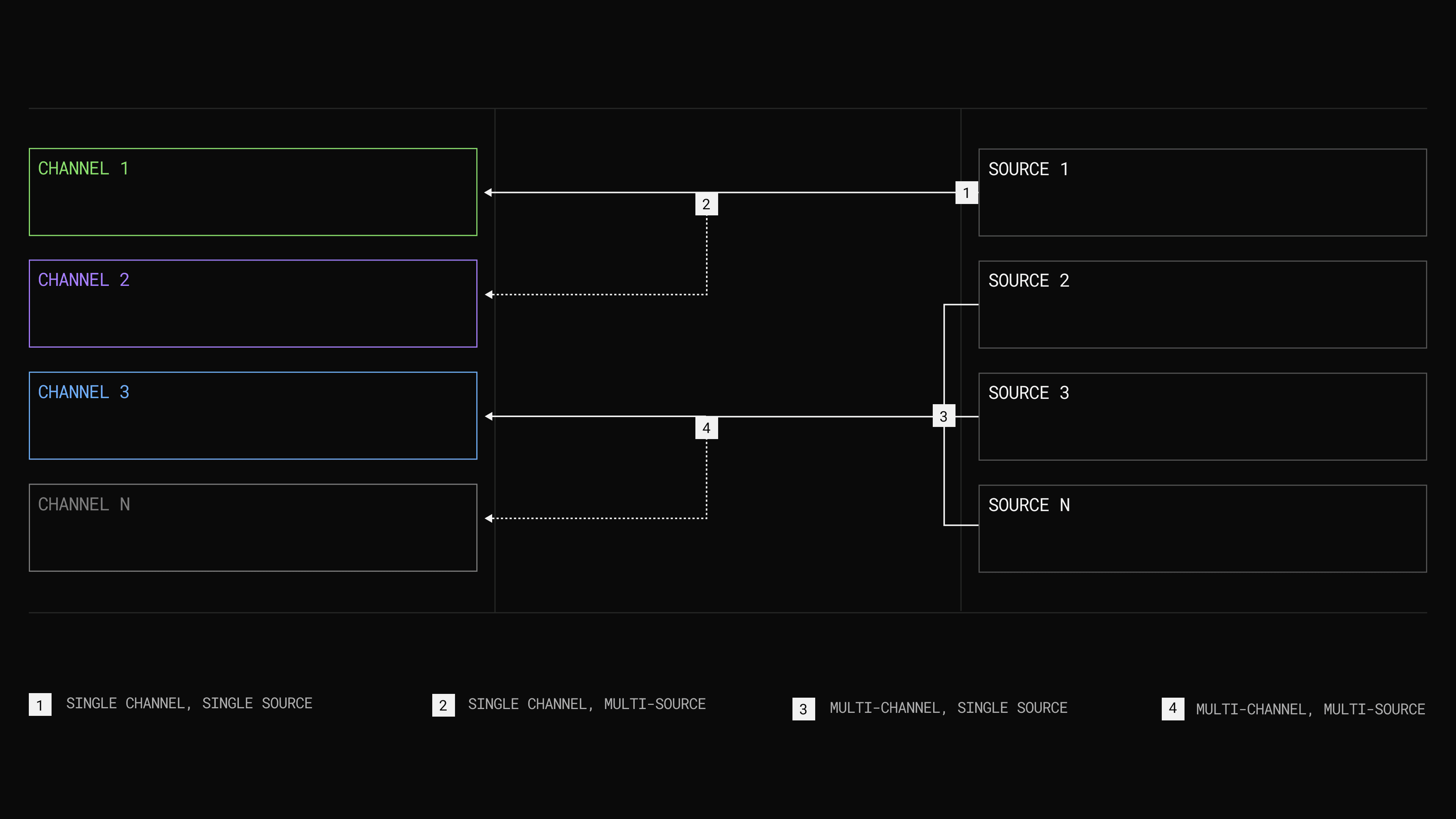
3. ReadLib: The ReadLib is an immutable Message Library that ensures query requests are emitted, delivered to the configured DVNs, and the responses are verified by the configured DVNs. Here are the ReadLib’s responsibilities:
- Reorg Protection: The stored cmdHash provides reorg protection, using the cmdHash to identify and reject responses (delivered by the DVNs) that correspond to an altered chain state, such as one resulting from a blockchain reorganization.
- Message Channel Enforcement: Ensures queries adhere to the correct messaging channels by mapping Channel IDs to their respective configurations of DVNs and executors. This mapping enforces strict routing, preventing queries from being processed by unauthorized or misconfigured infrastructure components.
- Deterministic Validation: Each DVN submits a cryptographic hash of the retrieved data i.e. payloadHash. The ReadLib enforces that all required DVNs and the minimum threshold of optional DVNs produce the same payloadHash – thus validating the query.
Processing Layer
Decentralized Verifier Network (DVN): DVNs compatible with lzRead are similar to LayerZero v2, with the exception that they are tasked with data retrieval i.e. fetching, computation, and validation. As the processing layer of lzRead, DVNs enable efficient and trustless execution of queries by fetching data from target chains or systems, performing optional computational transformations, and returning validated results to the source chain.
The DVN receives all the information it needs to perform its tasks through the following parameters:
- packetHeader: provides the context of the request and helps the DVN understand where the request originated (on the source chain) and where its result should be delivered (back to the source chain).
- cmd: what data to fetch and where to fetch it from. This includes which type of data (e.g., token price, account balance) on which contract, on which chain(s), across which time or time period.
- Options: Specifies optional parameters for data fetching, such as: Gas limits and Retry logic.
Each configured DVN is tasked with decoding the cmd, fetching and computing (optional) the data, and independently verifying the query. How it works is that once the cmd is received, DVNs decode it, i.e. resolve, to extract the query details and then fetch the corresponding data on the target chain(s) or external source(s). This may involve calling specific smart contract methods (e.g., view or pure functions) or reading public state variables. The flexibility of this approach allows DVNs to fetch a wide variety of data across both EVM and non-EVM chains.
lzRead Query Flow
Before building a read query, the application must configure the necessary components to ensure that the query can be processed securely and efficiently. This includes:
- Channel Configuration: Assigning Channel IDs to manage specific query types and their associated components, such as DVNs and executors.
- DVN Setup: Specifying the required and optional DVNs, as well as their thresholds for response validation.
Then, the application proceeds by building a query request according to the BQL semantics. In this example, the BQL semantics are specified in the EVMCallRequestV1 and EVMCallComputeV1 structures.
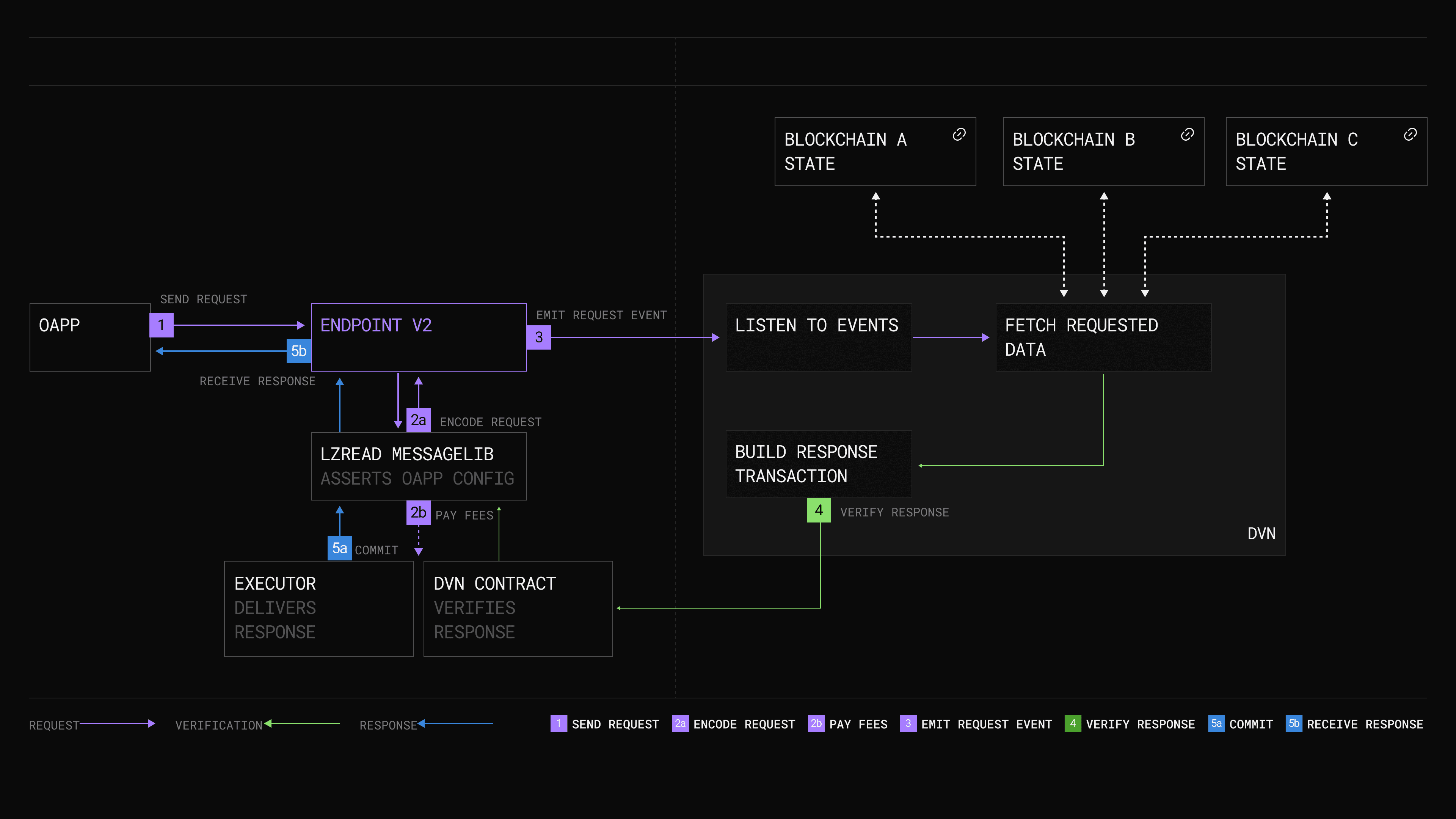
Now the application is ready to send a query request. Below, we detail the lifecycle of a lzRead query request and response:
- Send Request: The application encodes the query into a binary payload (cmd). The cmd is transmitted to the LayerZero endpoint via _lzSend, and routed through the appropriate channel as specified by the channelID.
- Fetch Data: Upon receiving the command, the configured DVNs decode the payload, extracting the target address, block number or timestamp, and calldata from the cmd parameter, and querying the target blockchain(s) to fetch the requested data.
- Compute: If compute instructions are included in the command, the DVNs perform additional transformations on the fetched data before returning it to the application. The compute stage involves:
- Mapping (lzMap): Each individual response is processed to extract or transform specific fields of interest. For example, the DVN might decode a Uniswap response to isolate a token price.
- Reducing (lzReduce): If multiple responses are aggregated, the DVN applies a reduction operation (e.g., calculating the average token price across chains).
- Validate: Once the data is fetched and any computations are performed, the DVNs validate the results. This validation is achieved through:
- PayloadHash Generation: Each DVN generates a cryptographic hash of the fetched or computed data (payloadHash), representing the final result of the query.
- Receive: Application receives the validated response.
Use Cases
Yield Streaming Vaults
Yield-bearing tokens, such as those issued under ERC-4626, are currently limited to single-chain environments due to the difficulty of attributing yield across chains. The challenge lies in managing local price per share (PPS) discrepancies. Existing solutions rely on offchain infrastructure and additional contracts, increasing complexity, cost, and attack surfaces. This restriction limits their use across multiple DeFi ecosystems, reducing composability for trading, lending, staking, and structured products.
lzRead can query totalAssets and totalSupply from the hub contract, while using compute to calculate the vault’s PPS. This can be received by vaults on all other chains – creating a global PPS. This enables use cases such as using yield-bearing tokens as collateral in lending protocols or creating derivative markets.
Plume announced their plans to use lzRead to power cross-chain yield streaming across 17 different networks.
Virtual DEXs
Decentralized exchanges on emerging or less liquid chains frequently encounter liquidity depth issues, leading to inefficient swaps and high slippage. Reliable price feeds are often unavailable, forcing developers to rely on third-party oracles, which introduce additional costs and centralization risks. Without accurate, onchain-derived pricing, protocols struggle with optimal trade execution.
lzRead enables permissionless asset pricing by retrieving prices directly from the deepest liquidity pools on any chain. DEXs can query the deepest pools using Uniswap TWAP oracles, for example, aggregate pricing data using lzMap and lzReduce, and deliver accurate, real-time valuations to their low-liquidity chain. This extends the concept of virtual AMMs, allowing applications to simulate deep liquidity without large capital pools, ultimately improving execution quality for swaps and lending markets.
Shadow NFTs
NFTs are typically restricted to the chain they were minted on, limiting their usability in applications such as games, marketplaces, and DeFi platforms on other chains. For instance, Bored Apes and Pudgy Penguins exist on Ethereum, whereas Ape Chain and Abstract operate independently, preventing direct NFT interoperability across ecosystems.
lzRead can be used for cross-chain NFT verification by retrieving ownership records directly from the source chain. When a user attempts to utilize an NFT in a game or application on another chain, an lzRead request fetches the NFT holder’s address from the originating chain. The response confirms whether the user attempting to authenticate is the rightful owner.
In fact, this solution is already built by ApeChain.
Cross-Chain Authentication
Decentralized identity solutions like World ID, Humanity Protocol’s DID, and ENS exist across multiple chains, creating fragmentation that complicates cross-chain authentication. Applications on new chains struggle to verify users without relying on centralized authentication providers, limiting accessibility and security.
Applications on any chain can use lzRead to query identity records from the chain where they are issued on. This can also extend to offchain sources whereby an identity is held in a trusted execution environment (TEE) and a verifiable API request and response is conducted using lzRead. This creates an avenue towards secure, decentralized, and permissionless identity verification across all blockchain ecosystems.
Conclusion
lzRead changes how smart contracts access and use data across chains. Instead of dealing with the limitations of fragmented onchain state with complicated, slow, and expensive solutions, lzRead provides a fast, cheap and secure method to pull real-time, historical, and even offchain data.
By leveraging BQL and LayerZero’s infrastructure, lzRead lets applications retrieve and compute data with minimal cost and latency while ensuring trustless verification. This means better pricing for DEXs, seamless use of yield-bearing tokens across chains, cross-chain NFT authentication, and decentralized identity verification—all of which can be done permissionlessly.
At its core, lzRead unlocks a new level of interoperability, giving developers the tools to build smarter, more connected applications. Whether it’s DeFi, NFTs, or authentication, accessing data from anywhere is no longer a bottleneck. Building without limits is now possible.
Appendix: lzRead vs. LayerZero
lzRead is part of the LayerZero protocol. However, the key difference is that while LayerZero allows one smart contract to send the same message to a smart contract on a different chain, lzRead allows one smart contract to send and receive a different message on the same chain.

This pattern can actually be accomplished through LayerZero with the ABA message pattern, a nested send call from Chain A to Chain B that sends back again to the source chain (A → B → A). However, as explained in the report, this message pattern incurs gas costs on each chain, and latency in two different directions. lzRead optimizes for this message pattern with a single-round of messaging, what can be referred to as the AA messaging pattern.
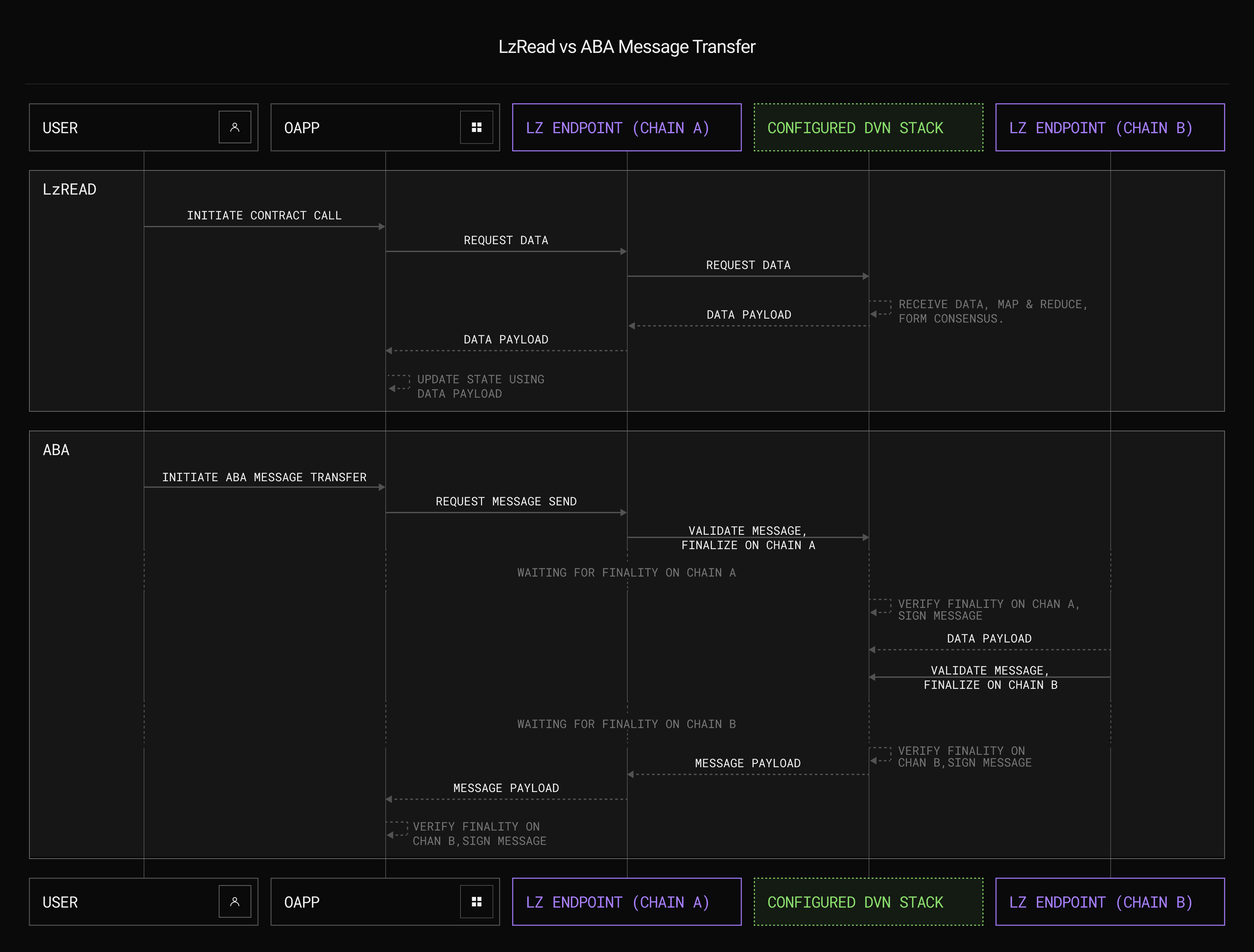
Resources
https://layerzero.network/publications/LayerZero_Whitepaper_V2.1.0.pdf
https://docs.layerzero.network/v2
https://vitalik.eth.limo/general/2023/06/20/deeperdive.html#what-does-a-cross-chain-proof-look-like
https://ethereum.org/en/developers/docs/standards/tokens/erc-4626/
https://docs.layerzero.network/v2/developers/evm/lzread/overview
About LayerZero
LayerZero is an interoperability protocol that connects blockchains, powering seamless omnichain apps and tokens. Used by teams like PayPal, Stargate, Ethena, USDT0, WBTC, and hundreds more, it ensures secure, censorship-resistant cross-chain messaging through immutable onchain endpoints, a flexible security stack, and a permissionless execution.